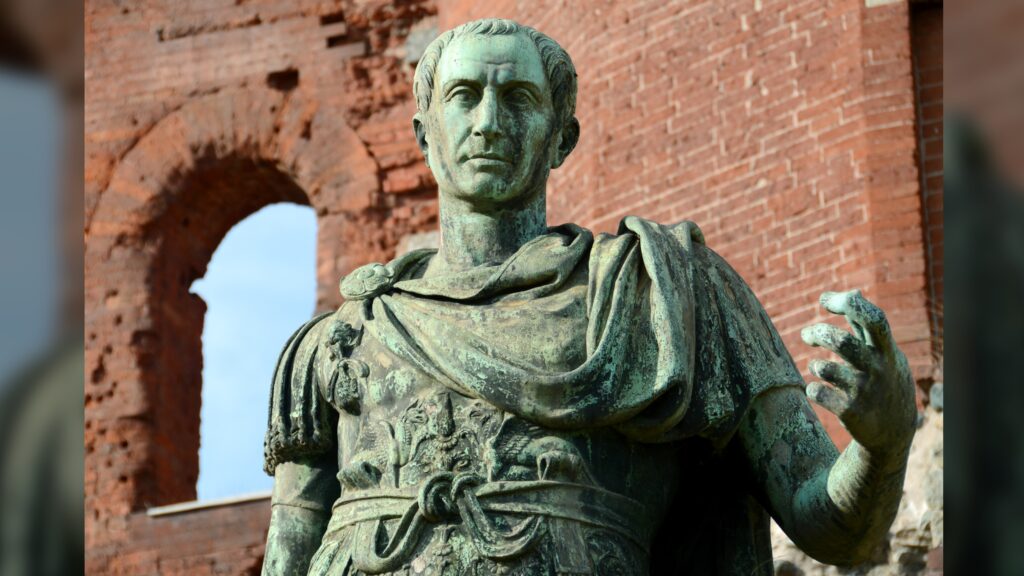
Encryption is an important part of any successful cybersecurity defense strategy. Not only do malicious actors have to capture the sensitive information, they have to crack the additional layer of security in order to get utility out of the stolen information. To compare it to a physical world example, encryption is like the massive vault inside the bank where the money is stored. Anyone who wants the money (sensitive information) not only has to break through the banks exterior defenses (firewalls, anti-malware, etc.), they have to crack the safe (encryption) before they can actually use what they came to steal. With that in mind, here is a simple Python encryption script (often called a Caesar cipher-hence the photo) that I have written using the ASCII table, which looks like this:
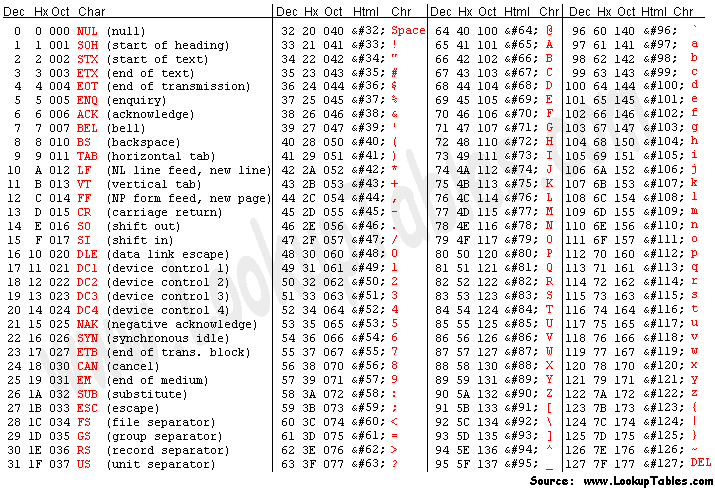
Here is my script:
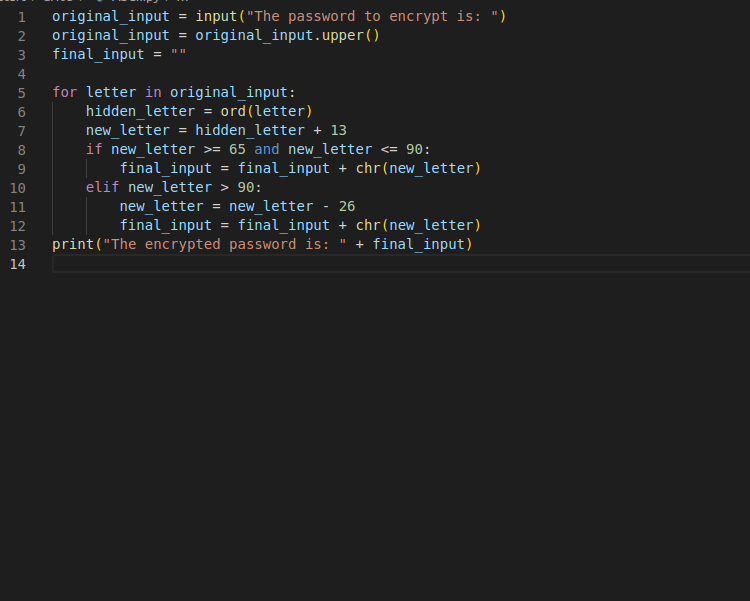
A breakdown of the script:
- The first three lines create two variables: the original and final input. The original input is the message we will be encrypting, and the final input is the encrypted message. Final input is left blank so we can create it later. The second line simply converts all letters into the upper case to simply the encryption function.
- The hashing function is a little bigger, so a line-by-line breakdown follows:
- The individual letters in the original input are run through the function, letter by letter
- The letters will be converted to a new variable, hidden_letter. This variable is going to be the number on the ASCII table that corresponds to the the letter/input (ex. ‘A’ is 65, ‘B’ is 66, ‘a’ is 97, and so on). ‘Ord(letter)’ is the function to call to convert the letter to the numerical value, with ‘letter’ as the input.
- The local variable new_letter is the hidden_letter variable (which is now actually a number!) with 13 added to the value.
- The next few lines are critical. Remember, all of our letters were converted to uppercase before the function. In the ASCII table, the 26 uppercase letters are numbers 65 through 90. If the value of new_letter is within that range, it is still an uppercase letter, which is what we want. The number will be run through the chr() function, which reverses the process that the ord() function performed earlier. We have added a new, encrypted letter to our “final_input” variable.
- If the value of new_letter is greater than 90, then new_letter will no longer be a letter. For this encryption, we are only using letters. Therefore, we will subtract 26 from the value of new_letter. What this does in practice is it causes new_letter to start back at the beginning of the alphabet when it goes past the letter ‘Z’, instead of continuing on to the special characters. From here, it’s the same process as before: we convert the number to a letter, and add it to our final_input variable.
- Once the function has run through and converted every letter, we will receive our new output, with a message. Time to view it in action:
It appears to work. There is one way to make sure that it works: if we feed the “encrypted password” as the input, it should return the original input of PatrickChristensen (in all capital letters, since the function capitalizes everything). Let’s check it out:
The encryption (and decryption) is successful. We get the same original (capitalized) input when we run the output back through the machine.